Paying out profits from the Taco Shop
In the previous tutorial we have learnt how to setup & interact with the LIGO CLI. Followed an implementation of a simple Taco Shop smart contract for our entrepreneur Pedro.
In this tutorial we will make sure Pedro has access to tokens that people have spent at his shop when buying tacos.
Analysing the Current Contract
taco-shop.jsligo
Purchase Price Formula
Pedro's Taco Shop contract currently enables customers to buy tacos, at a price based on a simple formula.
Designing a Payout Scheme
Pedro is a standalone business owner, and in our case, he does not have to split profits and earnings of the taco shop with anyone. So for the sake of simplicity, we will payout all the earned XTZ directly to Pedro right after a successful purchase.
This means that after all the purchase conditions of our contract are met, e.g., the correct amount is sent to the contract, we will not only decrease the supply of the individual purchased taco kind, but we will also transfer this amount in a subsequent transaction to Pedro's personal address.
Forging a Payout Transaction
Defining the Recipient
In order to send tokens, we will need a receiver address, which, in
our case, will be Pedro's personal account. Additionally we will wrap
the given address as a contract (unit)
, which represents either a
contract with no parameters, or an implicit account.
Would you like to learn more about addresses, contracts and operations in LIGO? Check out the LIGO cheat sheet
Adding the Transaction to the List of Output Operations
Now we can transfer the amount received by buy_taco
to Pedro's
ownerAddress
. We will do so by forging a transaction (unit, amount,
receiver)
within a list of operations returned at the end of our
contract.
Finalising the Contract
taco-shop.jsligo
Dry-run the Contract
To confirm that our contract is valid, we can dry-run it. As a result, we see a new operation in the list of returned operations to be executed subsequently.
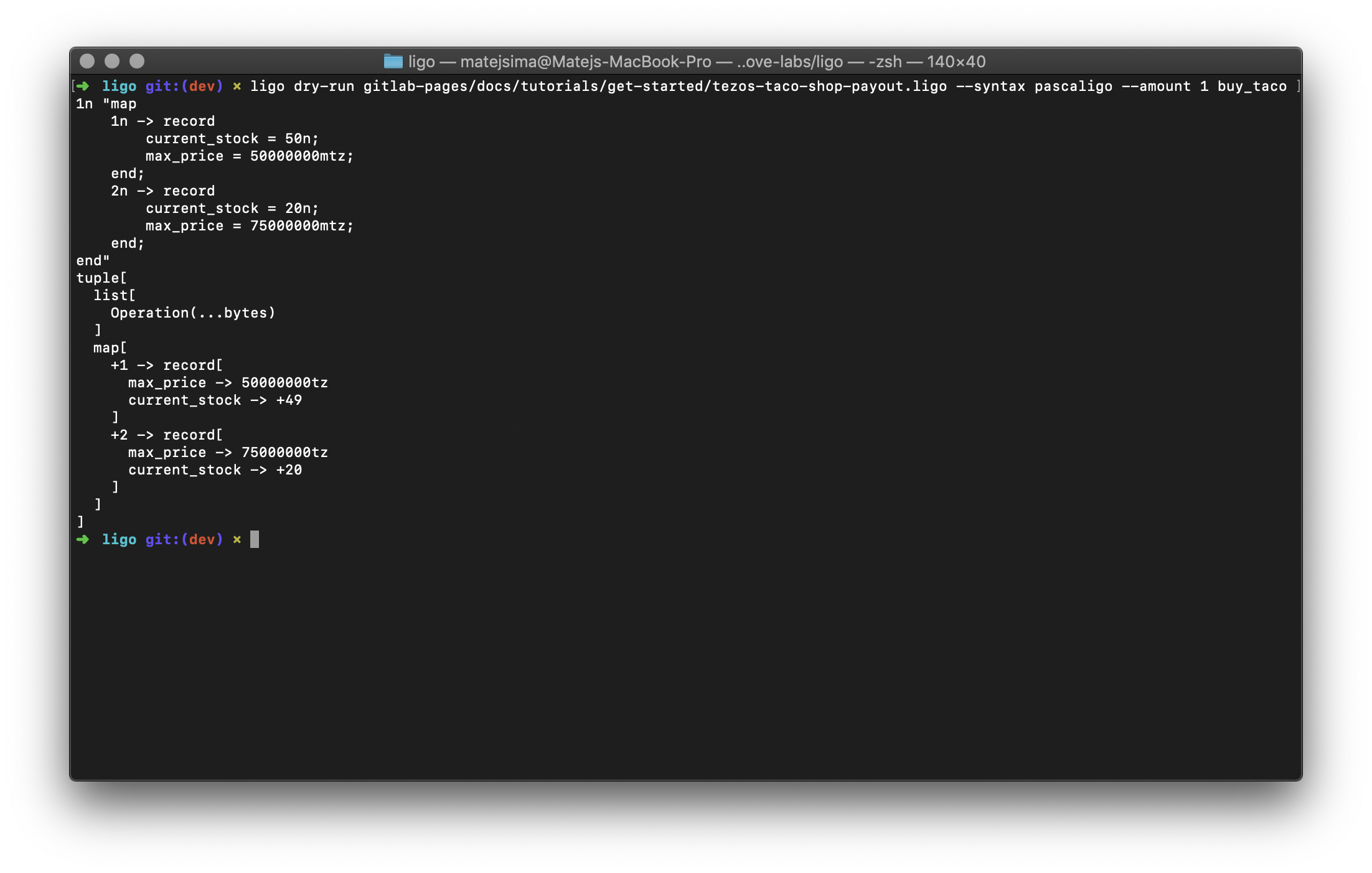
Done! Our tokens are no longer locked in the contract, and instead they are sent to Pedro's personal account/wallet.
👼 Bonus: Donating Part of the Profits
Because Pedro is a member of the Speciality Taco Association (STA), he
has decided to donate 10% of the earnings to the STA. We will just
add a donationAddress
to the contract, and compute a 10% donation
sum from each taco purchase.
This will result into two operations being subsequently executed on the blockchain:
- Donation transfer (10%)
- Pedro's profits (90%)